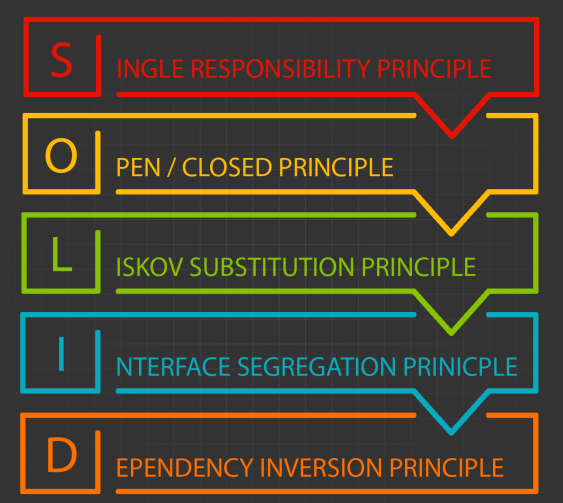
SOLID Principles
- What is Single Responsibility Principle (SRP)?
- What is Open Closed Principle (OCP)?
- What is Liskov Substitution Principle (LSP)?
- What is Interface Segregation Principle (ISP)?
- What is Dependency Inversion Principle (DIP)?
Stay tunned, I will cover this topic fast and simply put!
SOLID Principles and SRE
Introduction
The SOLID principles are a set of guidelines that help you write code that is more robust and easier to maintain. They were originally developed by the Gang of Four and have been widely adopted by many other developers as well. These principles help us build software that is more testable, reusable (and therefore cheaper), and maintainable over time.
Single Responsibility Principle
The Single Responsibility Principle (SRP) is one of the most important principles in software architecture. It states that each class should have a single responsibility and should not be responsible for more than one thing. The reason for this is that if you have multiple responsibilities, it’s harder to test your code and make sure it works correctly. You may also find yourself having to change multiple times because one part of your program has broken down or something unexpected happened somewhere else in the system. But if all classes have just one task or purpose, then they should be easier to understand by other developers on their team or by consumers who use them (i.e., end users).
Another reason why SRP encourages us as programmers/developers/architects/etc…to follow these guidelines includes making our lives easier when creating new features because we won’t need too much explaining about what each piece does before moving onto work at hand.
Open Closed Principle
The Open Closed Principle is a principle that states that a class should be open for extension, but closed for modification. This means that you want the class to be able to add new functionality without breaking existing code in your application. The most obvious way of implementing this is by using abstract classes or interfaces: they allow other classes (which extend them) to implement their methods without having any knowledge of how they work internally. In Java we can do something similar with traits: these are used in place of interfaces when only some parts need certain behaviour and others don’t; however, traits do not have access modifiers like interfaces do so they’re less flexible than their counterparts.
Liskov Substitution
Liskov Substitution Principle (LSP) is a contract between two classes, which states that an object of one class can be replaced by another object from the same or another class without affecting its behaviour. It means that if you have an object of class A and want to replace it with an instance of class B, then there should not be any changes in the behavior of your program. In other words:
public interface Vehicle {
public void drive();
}
public interface Car extends Vehicle {
public void accelerate();
}
Interface Segregation Principle
The interface should have a minimal and well-defined surface area. The client should not be able to affect the internal implementation of an API. This can be achieved by using interfaces, which define what is allowed to happen at each layer of the API (e.g., request/response). The interface should also be stable throughout its lifetime, meaning that it doesn’t change over time or in different situations (which might require different implementations). Also, you want your clients to know exactly how they interact with your system because this will help them avoid errors when interacting with your application in production environments where unexpected behavior could lead to downtime if left unchecked due to incompatibilities between systems’ implementations of certain features or behaviors required by certain clients’ needs when using those APIs for specific tasks such as order fulfillment operations like shipping orders etc..
Dependency Inversion Principle
Dependency inversion is a design principle used to increase the maintainability of software. It’s also known as the Dependency Injection Principle and was originally proposed by Bertrand Meyer in his book Object-Oriented Software construction: A Practical Approach. Dependency inversion is a form of decoupling, which means that you break up your code into smaller pieces that can be tested independently from each other (and therefore easier to test). The idea behind this is that if an object has dependencies on another object, you should separate those two classes so they don’t depend on each other anymore—it’s much easier for developers working on one part of the system to change their code without affecting another part. This separation also makes it easier for teams working together across different projects or areas within one company because they won’t have conflicting requirements between them; instead they’ll share goals based on common needs rather than trying constantly clash over technical details like whether something should be implemented using Poco::Any or Poco::Objects::Any .
One class with one job.
A class should have only one reason to change, and it should have only one reason to exist. A class’s purpose is typically defined by its business goals, but if your system has too many responsibilities or implementations, you risk creating more classes than necessary—and this can lead to confusing codebases and bloated architectures that make refactoring difficult.
Conclusion
In the end, we hope you’ve gained a better understanding of what it means to be a good SRE. We also want to encourage you to continue learning more about these principles, as well as other ways that can help your team scale and maintain quality software. The most important thing is for everyone on your team—whether it’s in person or remotely—to understand each others’ responsibilities and work together to keep things running smoothly!
Today’s tip is this video on Youtube:
Click on the Video to access the content: 👇👇👇👇👇
▸ The S.O.L.I.D Principles in Pictures: https://medium.com/backticks-tildes/the-s-o-l-i-d-principles-in-pictures-b34ce2f1e898
Please, follow our social networks:
Thank You and until the next one! 😉👍
Published on Jul 25, 2022 by Vinicius Moll